Git操作文档
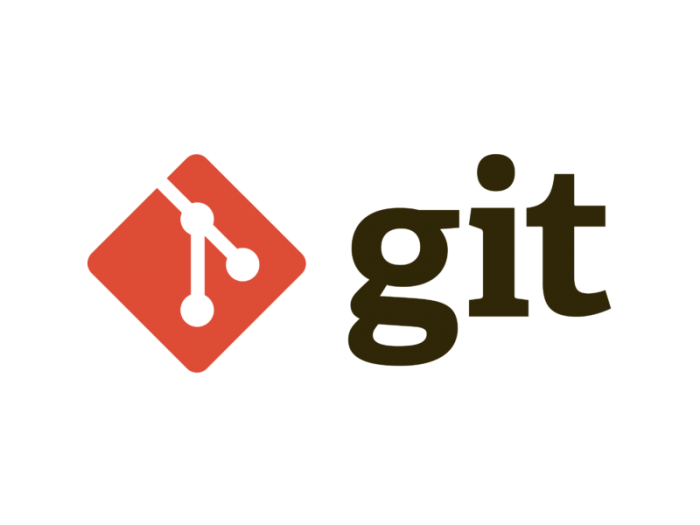
Git操作文档
吴华锦类型 | 说明 |
---|---|
feat |
新特性 |
fix(scope) |
修复 scope 中的 Bug |
feat!:/feat(scope)!: |
breaking change / 重构 API |
build |
变更影响的是构建系统或者外部依赖 (如: gulp , npm ) |
ci |
修改了 CI 配置文件或脚本 (如: Github Action , Travis ) |
chore |
【重要】 变更不影响源代码或测试(如更新了辅助工具、库等) |
docs |
只修改了文档 |
feat |
【重要】 一个新特性 |
fix |
【重要】 修复了一个 Bug |
perf |
增强性能的代码变更 |
refactor |
并非修复 Bug 或添加新特性的代码变更 |
revert |
回退代码 |
style |
变更不影响一些有意义的代码 (如: 删除空格、格式化代码、添加分号等) |
test |
添加测试代码或修正已有的测试 |
提交(Commit)
查看HEAD上最近一次的提交(commit)
1 | git show |
修改提交信息(commit message)
1 | git commit --amend --only |
修改提交的用户名和邮箱
1 | git commit --amend --author 'new authorname email' |
从一个提交移除一个文件
1 | git checkout HEAD^ myfile |
删除最后一次提交
1 | git reset HEAD^ --hard |
删除任意提交
1 | git rebase --onto SHA1_OF_BAD_COMMIT^ SHA1_OF_BAD_COMMIT |
强推到远端
1 | git push -f origin my-branch |
硬重置(hard reset)后,找回内容
1 | git reflog |
暂存(Staging)
把暂存(staging)的内容添加到上一次的提交
1 | git commit --amend |
只暂存一个新文件的一部分,不是全部
1 | git add --patch[-p] filename.x |
把在一个文件里的变化(changes)加到两个提交里
1 | git add -p |
把暂存的内容变成未暂存,把未暂存的内容暂存
1 | git commit -m "WIP" |
未暂存(Unstaged)
把未暂存(unstaged)的内容移动到一个新分支
1 | git checkout -b my-branch |
把未暂存的内容移动到另一个已存在的分支
1 | git stash |
丢弃本地未提交的变化(uncommitted changes)
1 | // one commit |
丢弃某些未暂存的内容
1 | git checkout -p |
分支(Branches)
从错误的分支拉取了内容,或把内容推送到了错误的分支
1 | git reflog |
丢弃本地的提交,以便本地分支与远程保持一致
1 | git status |
需要提交到一个新分支,但错误的提交到了main
1 | git branch my-branch |
保留来自另一个ref-ish的整体文件
1 | git add -A && git commit -m "commit message" |
删除上游(upstream)分支被删除了的本地分支
1 | git fetch -p |
不小心删除了分支
1 | git checkout -b my-branch |
删除一个远程分支
1 | git push origin --delete my-branch |
删除一个本地分支
1 | git branch -D my-branch |
从别人正在工作的远程分支迁出(checkout)一个分支
1 | git fetch --all |
Rebasing和合并(Merging)
撤销rebase/merge
1 | git reset --hard ORIG_HEAD |
已经rebase,但不想强推(force push)
1 | git checkout my-branch |
需要组合(combine)几个提交
1 | git reset --soft main |
安全合并(merging)策略
1 | git merge --no-ff --no-commit my-branch |
将一个分支合并成一个提交
1 | git merge --squash my-branch |
组合(combine)未推的提交
1 | git rebase -i @{u} |
检查是否分支上的所有提交都已经合并
1 | git log --graph --left-right --cherry-pick --outline HEAD...feature/120-on-scroll |
暂存所有改动
1 | git stash |
暂存指定文件
1 | git stash push working-directory-path/filename.ext |
暂存时记录消息
1 | git stash save <message> |
使用某个指定暂存
1 | git stash list |
暂存时保留未暂存的内容
1 | git stash create |
杂项
克隆所有子模块
1 | git clone --recursive git://github.com/foo/bar.git |
删除标签(tag)
1 | git tag -d <tag_name> |
恢复已删除标签
1 | git fsck --uncreachable | grep tag |
跟踪文件(Tracking Files)
只改变一个文件名字,不修改内容
1 | git mv --force myfile MyFile |
从Git删除一个文件,但保留该文件
1 | git rm --cached log.txt |
配置(Configuration)
缓存一个仓库(repository)的用户名和密码
1 | git config --global credential.helper cache |
修改远程分支名
1 | git branch -m oldbranch newbranch |
评论
匿名评论隐私政策